Replace your Django database with PlanetScale
Learn how to swap out an existing Django database and replace it with a PlanetScale database.
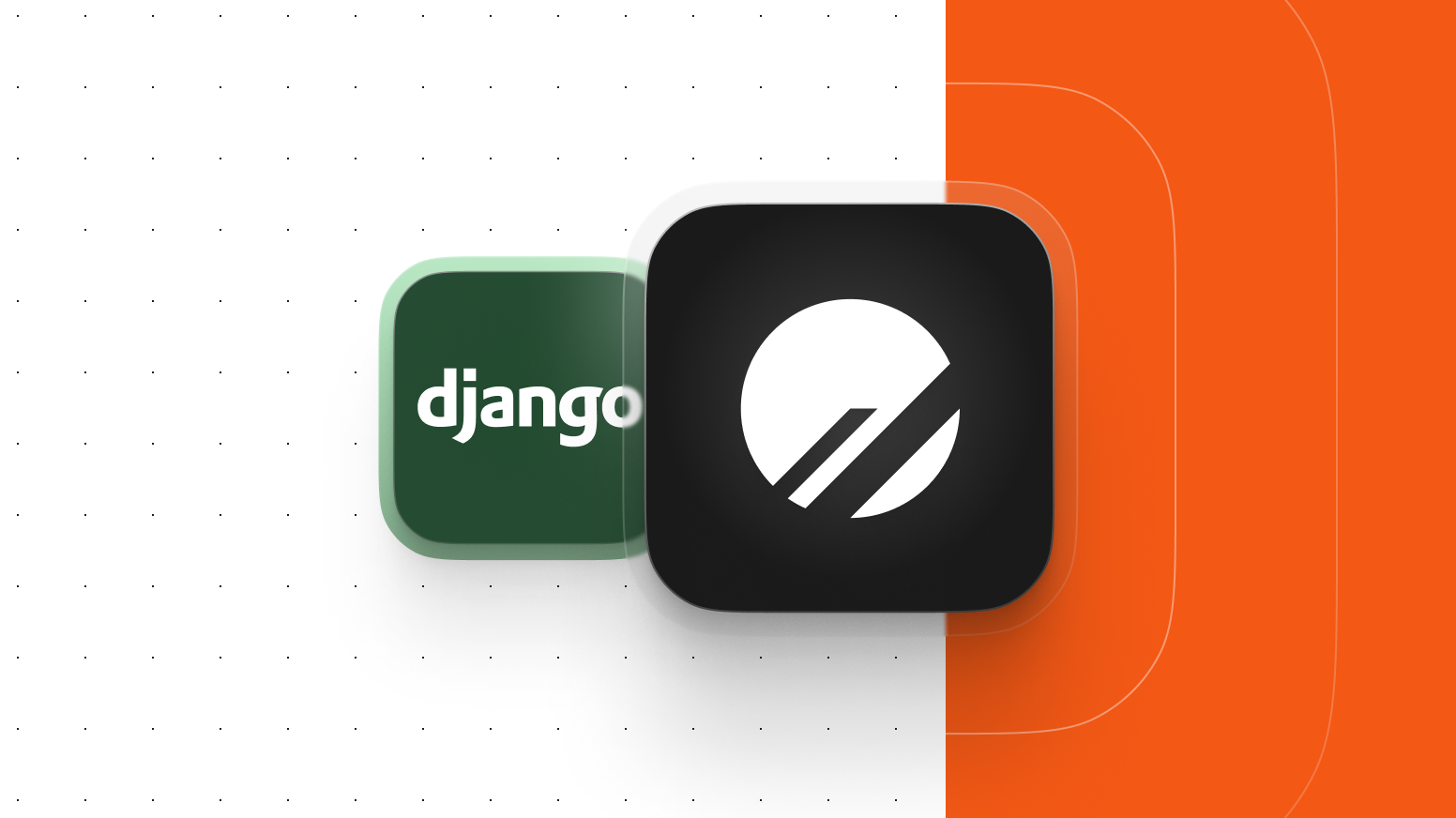
Almost every deployed backend web server encounters scalability challenges at some point.
Often, they appear in the form of slow database queries resulting from an increased number of system users. More users generally make for increased reads and writes, which can be especially detrimental to databases deployed on bare metal servers with concrete capacities and workloads. The traditional answer has been to upgrade the servers.
However, the perpetual process of trying to optimize database capacities can take up a lot of time and focus.
PlanetScale, a MySQL-compatible database, aims to solve that problem. PlanetScale is built on Vitess, the technology that was built to scale YouTube in its early days. In addition to scalability, you also get:
- An intuitive developer workflow with branching and deploy requests for your database
- In-dashboard advanced query monitoring
- The ability to instantly revert schema changes
- And more
In this article, you’ll learn how to replace your Django application's database with PlanetScale. This example uses dbsqlite3
, but can be extended to most other options.
Setting up the database
PlanetScale is a MySQL-compatible database. This means that it manages all of the scaling concerns that have long burdened developers. As a developer who uses PlanetScale, you no longer have to worry about provisioning new instances or increasing database capacity to keep pace with workloads.
With PlanetScale, you only need to sign up, create your database via the included dashboard or CLI, create a database branch, and connect to it. This demonstration will use the PlanetScale CLI, but you can also use the dashboard if you prefer.
This blog post is over a year old and may be out of date.
Before following this tutorial, you need to set up a basic Django database application with SQLite 3. Clone this demo repository, which will be used throughout the tutorial.
You need to generate a secret key and add it in server/backend/settings.py
.
Let’s create your database.
Go to the PlanetScale dashboard and click "New database" > "Create new database".
Give your database a name and select the region closest to you or your application.
This will spin up your new database with a development branch called
main
.This is an isolated development database that you can use to apply your schema, which we’ll do throughout this tutorial. Once finished, you can promote it to production, where it will be highly available, protected from direct schema changes, and backed up daily.
You can now connect the database on the
main
branch to your Django application. Click "Connect" > select "Django" from the "Connect with" dropdown > and if the password is blurred, click "New password".Copy these credentials or leave this tab open, as you’ll need to use them soon.
Connecting to PlanetScale in Django
If you haven’t already, clone the sample repository, and follow the instructions in the README
to get it running.
In server/backend/settings.py
file (or main.py
), replace the imports section up top with:
import os
import MySQLdb
import pymysql
pymysql.install_as_MySQLdb()
You’ll also need to install the python-dotenv
, mysqlclient
, and PyMySQL
packages to enable connecting to your database for local development:
pipenv install python-dotenv mysqlclient PyMySQL
Next, open server/backend/_init_.py
and add the following:
import pymysql
pymysql.install_as_MySQLdb()
Now, open server/manage.py
and add the following after the imports to reference your .env
file:
from dotenv import load_dotenv
load_dotenv()
Finally, if you haven’t already, create a .env
file to store all of your database connection credentials.
You can now replace the sqlite3 database in the Django application with the PlanetScale connection credentials.
Since PlanetScale doesn’t recommend foreign key constraints, you’ll need to set up the custom PlanetScale database wrapper for Django if you'd like to proceed without foreign key constraints. This will disable the foreign key syntax for Django migrations.
If you'd prefer to enforce referential integrity at the database level, you can still do so on PlanetScale. Head to your database settings page and enable foreign key constraint support. You can then use the standard, django.db.backends.mysql
database engine.
To do this, you can pull the repo directly from GitHub and include it in the server directory:
git clone https://github.com/planetscale/django_psdb_engine.git
Your settings.py
file should now include the PlanetScale database wrapper as shown below:
DATABASES = {
'default': {
'ENGINE': 'django_psdb_engine',
'NAME': os.environ.get('DB_NAME'),
'HOST': os.environ.get('DB_HOST'),
'PORT': os.environ.get('DB_PORT'),
'USER': os.environ.get('DB_USER'),
'PASSWORD': os.environ.get('DB_PASSWORD'),
'OPTIONS': {'ssl': {'ca': os.environ.get('MYSQL_ATTR_SSL_CA')}}
}
}
Next, paste your PlanetScale credentials that you copied earlier into your .env
file:
DB_HOST=xxxxxxxxxxx.eu-west-3.psdb.cloud
DB_PORT=3306
DB_DATABASE=demo-database
DB_USER=xxxxxxxxxxxxxx
DB_PASSWORD=pscale_pw_xxxxxxxxxxxxxxxxxxxxxxxxxxx
MYSQL_ATTR_SSL_CA=/etc/ssl/cert.pem
Your application should now be connected to your PlanetScale development branch, main
. Let’s run some migrations to add the schema.
Run Django migrations
You can now run your Django migrations as usual with:
python manage.py migrate
This also runs the default Django migrations.
This adds the schema to your PlanetScale database branch. You can double check that it was adding in the PlanetScale dashboard by clicking "Branches" > main
> "Schema".
Restart the application with:
python manage.py runserver
Add data
You’re now ready to test your demo application. Click the "Add your details" button, fill out the form, and click "Save".
The user information you just added was written to the PlanetScale database, which is being queried to output the data in your application, as shown below.
Promote to production
When you're finished making development changes, you can promote this main
branch to production. Go to the main
branch overview page in the dashboard, click "Promote a branch to production", make sure the "safe migrations" toggle is enabled, and click "Promote branch".
Your production branch is protected from direct schema changes (as long as you have safe migrations enabled) and highly available. Whenever you need to make another schema change, you’ll create a new development branch off of production, make your schema changes there, and open up a deploy request to merge those changes into your production database.
Conclusion
As you’ve seen first-hand, the PlanetScale workflow is straightforward and enables you to quickly provision new database environments for both development and production. You’ve also seen the ease with which you can replace an existing database with PlanetScale.